VMware vCloud Usage Meter – Product Credential Monitoring
vCloud Usage Meter is a virtual appliance installed on vCenter Server. vCloud Usage Meter collects consumption data and generates reports on supported products. This product can be used by customers included in the VMware Cloud Provider Program. In this article, I will explain how we can monitor the relevant service credentials in the products in vCloud Usage Meter with Python. After collecting the relevant error data on Python, we will be notified from the Slack channel as in my previous articles. Our use-case here is to receive notification of this situation as soon as possible in order not to affect Usage Meter usage reports in credential changes (password update, etc.) that will cause collection errors in related products. To do this, it is possible to receive notifications via e-mail about Product Collection Errors in the product. However, if you want to send notifications to the relevant teams via Slack, I will talk about how we can do this below.
You can access the VMware vCloud Usage Meter API document here.
After we connect Usage Meter with the following variables, we will check the status of the accounts in the all product list and send any errors to the Slack channel.
import requests
import json
from slack_sdk.webhook import WebhookClient
vcloudUM_URL = "https://vcloud-um.vmbro.local/login" # Usage Meter URL for Slack hyperlinked message
slack_emoji = ":warning:" # Slack Emoji
slackURL = 'https://hooks.slack.com/services/TTTTT535353' # Slack channel webhook URL
url = "https://vcloud-um.vmbro.local:443/api/v1/" # Usage Meter URL
UMUsername = "usagemeter" # Usage Meter username
UMPassword = "Password" # Usage Meter password
loginUrl = url + "login"
errorState = False
Below we create a session with Usage Meter URL and account information.
headers = {
"Content-Type": "application/json"
}
payload = {
"user": f"{UMUsername}",
"password": f"{UMPassword}"
}
response = requests.post(loginUrl, json=payload, headers=headers, verify=False)
sessionID = response.json()["sessionid"]
After obtaining the Session information, we can see the entire product list with the ProductURL.
productResponseJson = requests.get(productUrl, headers=headers, verify=False)
productResponse = productResponseJson.json()
formatted_json = json.dumps(productResponseJson.json(), indent=4)
print(formatted_json)
products = json.loads(formatted_json)
Here you can see the sample json output specific to the Aria Operations product. In the values under Status, we can see the error status in the related vROPS product.
[
{
"lastChanged": 1695226504400,
"vcHosts": [
"vcenter.vmbro.local"
],
"active": true,
"fullName": "VMware Aria Operations",
"inventory": [
{
"id": 4,
"vc_instance_uuid": "7332bffa-3022-4611-f33c-caffd0ffcf3dc"
}
],
"version": "8.12.1",
"port": 443,
"host": "ariaops-manager.vmbro.local",
"metered": true,
"id": 5,
"user": "usage-meter.svc",
"productType": "VROPS",
"status": {
"code": "Err",
"level": "Err",
"lastChanged": 16336512838,
"text": "Credential verification failure",
"statusCode": "PM_TEST_CRED"
}
}
]
Finally, in the for loop, we compare the statusCode values of all products respectively and send them to the sendSlack() function to receive notifications on the Slack channel for products containing errors.
for product in products:
if product["status"]["statusCode"] != "COLLECT_OK":
productType = product["productType"]
productUser = product["user"]
productInstance = product["host"]
productStatusText = product["status"]["text"]
productStatusCode = product["status"]["statusCode"]
sendSlack(productType, productUser, productInstance, productStatusText, productStatusCode)
After the sendSlack() function is executed, you will receive a notification in the Slack channel as follows. You can schedule the script as you wish and regularly monitor your Usage Meter products.
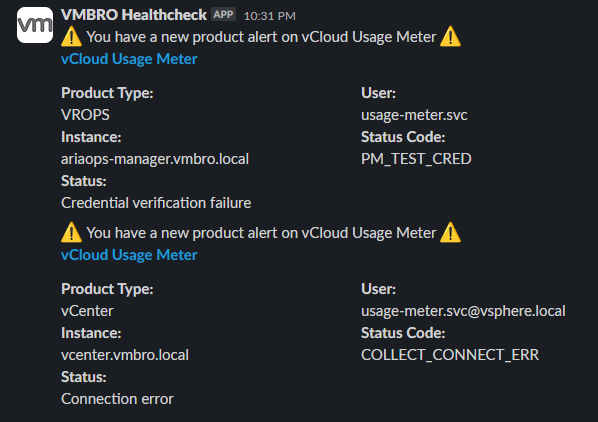
You can access the script used in the article from my Github profile.